前回までは画面の作成を行っていました!
今回は作成した画面に対して、C#のメイン処理を記載していきます!
今回はこのアプリの主役と言っていい変動するボタンの生成をC#上で実装していきます。
前回までは下記リンクをご参照ください!
ボタンの作成の基本
基本的にボタン等に関してはxamlファイルに直接書き込んでいきます。例えばこんな感じで記載すると
<Button Name="sampleButton" Content="test" Grid.Column="1" Grid.Row="1"/>
画面にボタンが表示されます。これにc#のコード上で実行する内容を記載すれば動作します。
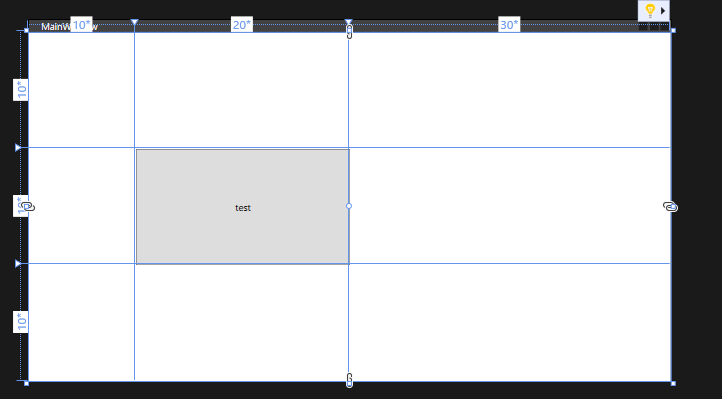
通常のだとこれでいいのですが、これだと変動するボタン数には対応できません。。。
C#のコード上でボタンを生成する
そのため条件によってボタン数を変動するコードをC#上で記載していきます!
//ボタン用の配列を作成します
//今回はファイル数を読み込めば上限が決められるので一旦箱だけ作成します
private Button[] WorkPanelButton;
//ボタンのコンフィグ+配置設定
private void createButtonConfig(String FileName,int i)
{
//各種ボタンの設定
this.WorkPanelButton[i] = new Button(); //ボタンの生成
WorkPanelButton[i].Content = FileName; //Contextの設定(Filenameは外部から入力)
WorkPanelButton[i].Name = "btn_" + i.ToString(); //動作させるためにName設定
WorkPanelButton[i].Tag = i.ToString(); //Tag設定
WorkPanelButton[i].Width = 200; //ボタンの長さ設定
WorkPanelButton[i].Height = 50; //ボタンの高さ設定
WorkPanelButton[i].Click += (sender, e) => ButtonDynamicEvent(sender); //イベントハンドラ設定(クリック動作させるよ!)
//スタックパネル(横置き)にボタン追加
AutoStackPanel.Children.Add(WorkPanelButton[i]); //べつでAutoStackPanelというレイアウトを用意して横置きにできるようにしています
}
これをfor文などで回すと回した分だけボタンが生成されます
実際に動作してみる
今回の要素を使用して動作確認してみました全体のソースはこんな感じです
[MainWindow.xaml]
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="10*" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="10*" />
</Grid.ColumnDefinitions>
<ScrollViewer HorizontalScrollBarVisibility="Auto" Grid.Column="0" Grid.Row="0">
<StackPanel x:Name="xamlMainStackPanel" />
</ScrollViewer>
</Grid>
</Window>
[MainWindow.xaml.cs]
using System;
using System.Windows;
using System.IO;
using System.Windows.Controls;
using System.Windows.Media;
using System.Diagnostics;
namespace WpfApp1
{
/// <summary>
/// MainWindow.xaml の相互作用ロジック
/// </summary>
public partial class MainWindow : Window
{
private Button[] WorkPanelButton;
public MainWindow()
{
InitializeComponent();
sampleButtonCreate(10);
}
private void sampleButtonCreate(int i)
{
int count = 0;
this.WorkPanelButton = new Button[i];
while (i > count)
{
createButtonConfig("test", count);
count++;
}
}
private void createButtonConfig(String FileName, int i)
{
//各種ボタンの設定
this.WorkPanelButton[i] = new Button();
WorkPanelButton[i].Content = "btn_" + i.ToString();
WorkPanelButton[i].Name = "btn_" + i.ToString();
WorkPanelButton[i].Tag = i.ToString();
WorkPanelButton[i].Width = 200;
WorkPanelButton[i].Height = 50;
WorkPanelButton[i].Click += (sender, e) => ButtonDynamicEvent(sender);
//次回レイアウトの説明をするので今回は直接xamlに配置しています
xamlMainStackPanel.Children.Add(WorkPanelButton[i]);
}
//自動生成されたボタンのクリックイベント
private void ButtonDynamicEvent(object sender)
{
int TagNo = Int32.Parse(((Button)sender).Tag.ToString());
//ゲーム起動
//ここに何をするか動作を入れる
}
}
}
実行してみるとこんな感じで出力されます
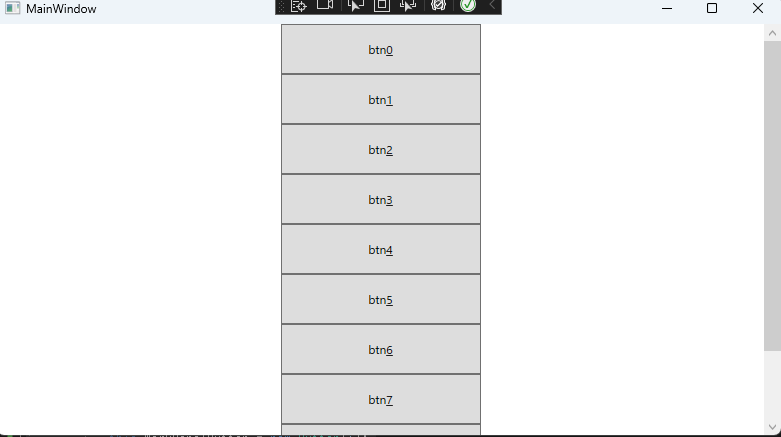
コメント